<目次>
(1) ASP.NETでビュー(View)に複数のモデル(Model)を連携する方法
(1-1) やりたい事の整理
(1-2) ViewModelを使った複数モデルの連携
(1-3) ViewModel導入手順
(1) ASP.NETでビュー(View)に複数のモデル(Model)を連携する方法
(1-1) やりたい事の整理
(1-2) ViewModelを使った複数モデルの連携
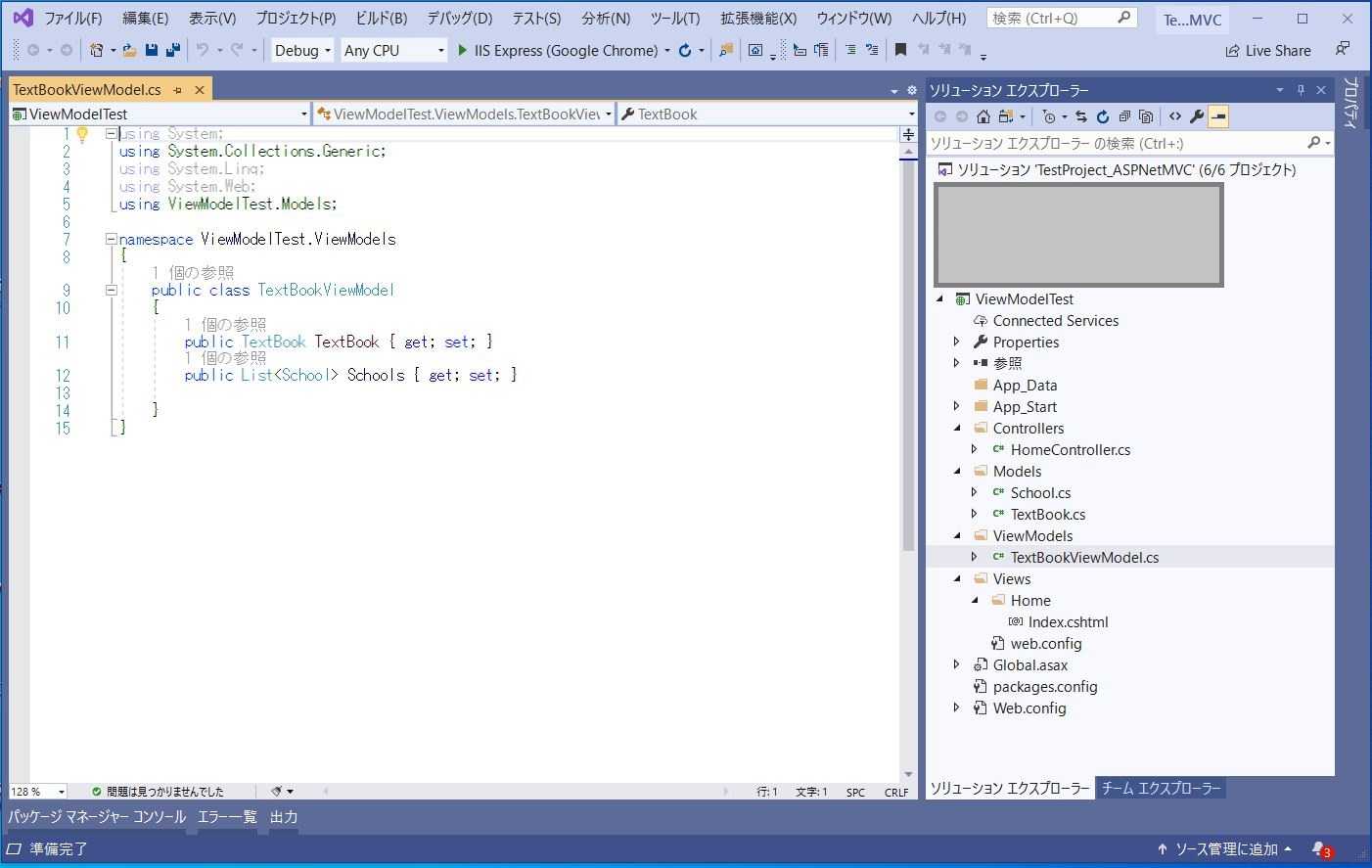
(1-3) ViewModel導入手順
●STEP0:モデル(Model)の準備
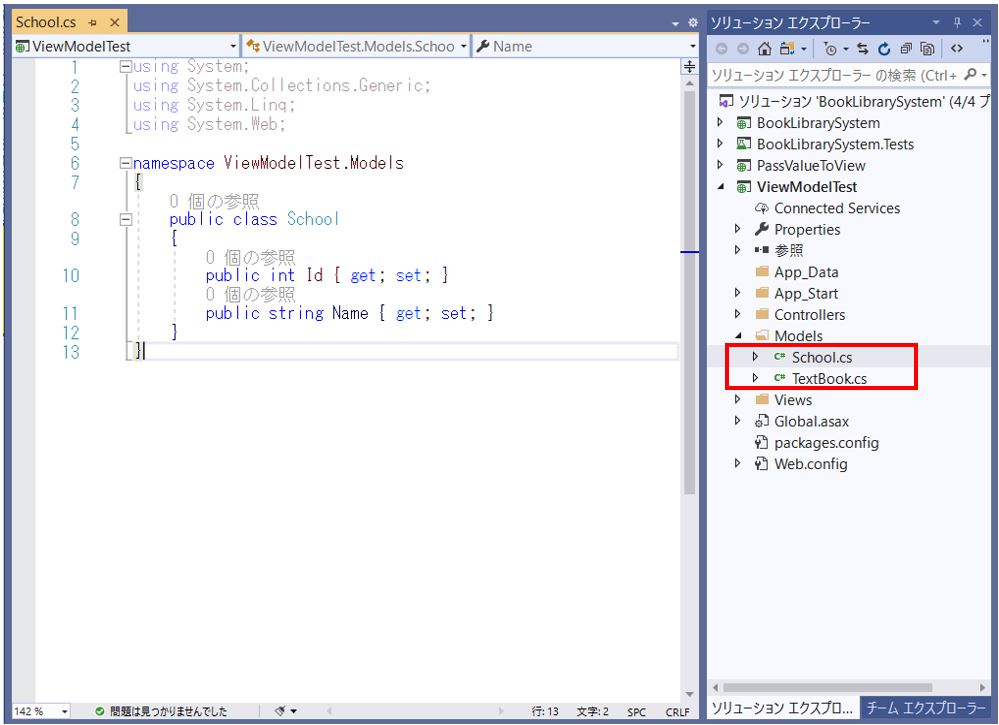
(School)
using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace ViewModelTest.Models { public class School { public int Id { get; set; } public string Name { get; set; } } }
(TextBook)
using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace ViewModelTest.Models { public class TextBook { public int Id { get; set; } public string Name { get; set; } } }
●STEP1:ViewModelの作成
using System; using System.Collections.Generic; using System.Linq; using System.Web; using ViewModelTest.Models; namespace ViewModelTest.ViewModels { public class TextBookViewModel { public TextBook TextBook { get; set; } public List Schools { get; set; } } }
(図134)
●STEP2:コントローラー(Controller)の追記
コントローラー(Controller)では、ビュー(View)に引き渡す値として、元々のモデル「TextBook」ではなく、今回用意したViewModelである「TextBookViewModel」を連携するように変更します。
・変更前
(図135)①
・変更後
(図135)②
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using ViewModelTest.Models; using ViewModelTest.ViewModels; namespace BookLibrarySystem.Controllers { public class HomeController : Controller { // GET: TextBooks/Trend public ActionResult Index() { var textbook1 = new TextBook() { Name = "理科" }; //# 追記 var schools = new List<School> { new School {Name = "School-1"}, new School {Name = "School-2"}, new School {Name = "School-3"} }; //# 追記 var viewModel = new TextBookViewModel { TextBook = textbook1, Schools = schools }; //# 修正:引数を変更 return View(viewModel); } } }
●STEP3:ビュー(View)の追記
@model ViewModelTest.ViewModels.TextBookViewModel
・また、次の記述でモデル内のデータにアクセスします。
<!-- 方法1 --> <h2>教科書名:@Model.TextBook.Name</h2> <h2>学校名1:@Model.Schools[0].Name</h2> <h2>学校名2:@Model.Schools[1].Name</h2> <h2>学校名3:@Model.Schools[2].Name</h2>
(図136)
(サンプル)
@model ViewModelTest.ViewModels.TextBookViewModel <!-- 方法1 --> <h2>教科書名:@Model.TextBook.Name</h2> <h2>学校名1:@Model.Schools[0].Name</h2> <h2>学校名2:@Model.Schools[1].Name</h2> <h2>学校名3:@Model.Schools[2].Name</h2> @{ ViewBag.Title = "Home Index"; } <div class="jumbotron"> <h3>本文</h3> <p class="lead">あああああああ</p> </div>
●STEP4:結果確認
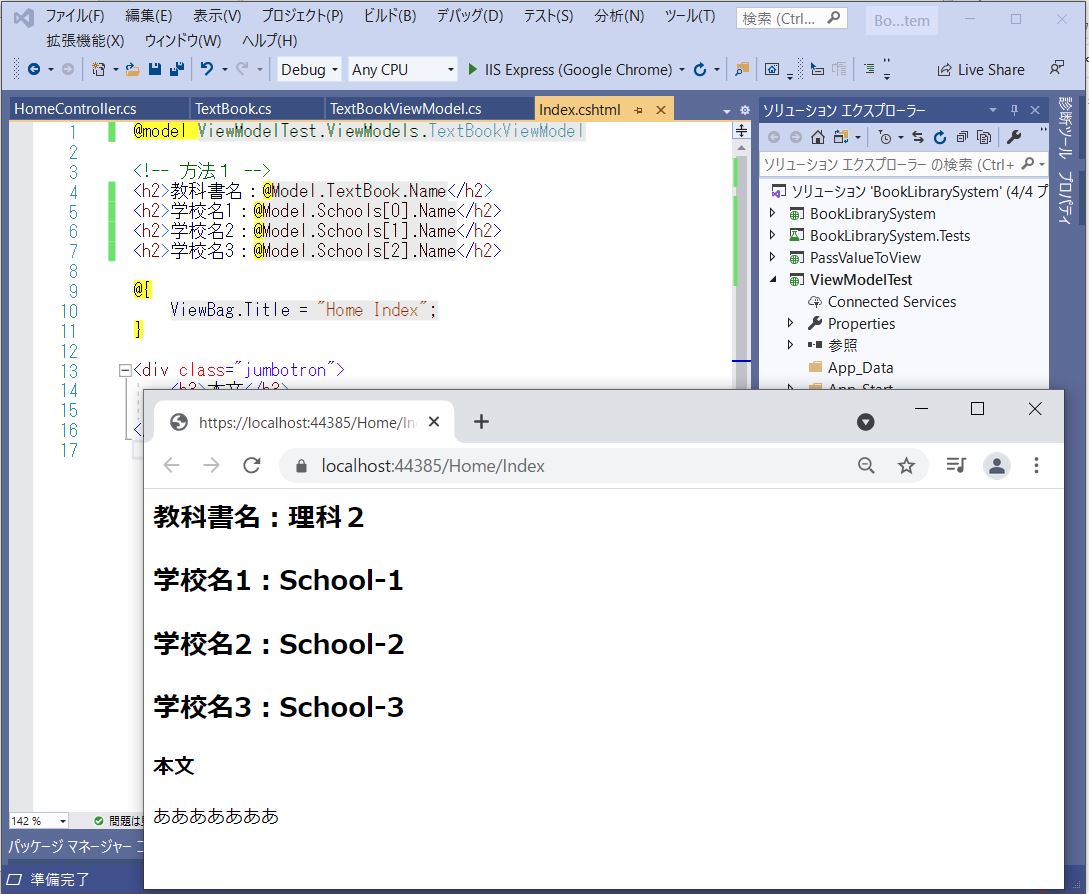
[…] ASP.NETでビュー(View)に複数のモデル(Model)を連携する方法 […]