<目次>
(1) Tensorflowにおけるplaceholderとは?v2.0以降での置き換え
(1-1) 「placeholder」とは
(1-2) サンプルプログラム
(1-3) Variableとの違いは?
(1-4) TensorFlow 2.0以降における置きかえ
(1) Tensorflowにおけるplaceholderとは?v2.0以降での置き換え
(1-1) 「placeholder」とは
import os os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3' import tensorflow.compat.v1 as tf tf.disable_v2_behavior() # placeholderを使って、先に関数を組み立て # この時点ではまだxには値は代入されていない # またxのサイズはNoneを指定し、後からdictionaryで任意のサイズを指定可能に x = tf.placeholder("float", None) y = x * 3 with tf.Session() as session: # 関数「y = x * 2」にx = 1,2,3を代入し、それぞれの結果を表示 # ⇒グラフの一部の領域に対して計算を実行できる result = session.run(y, feed_dict={x: [1, 2, 3]}) print(result)
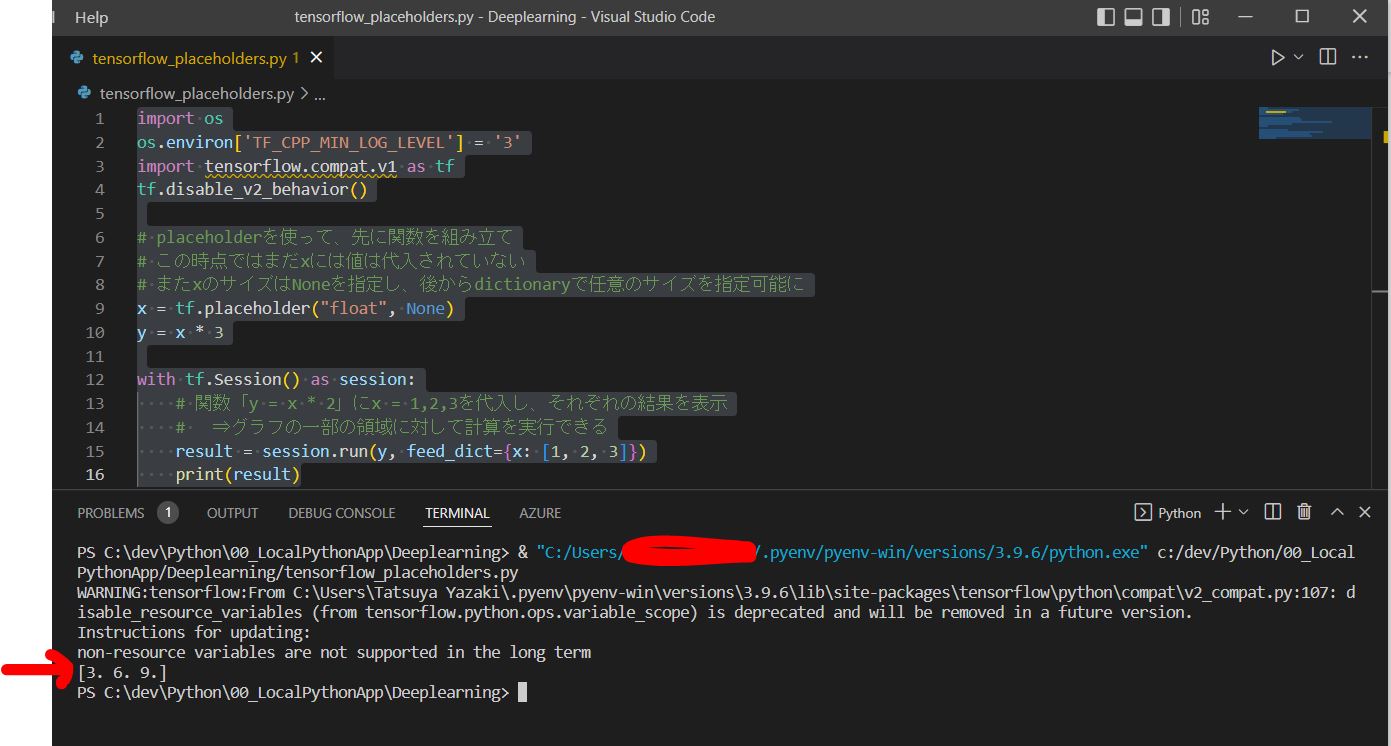
(1-2) 「placeholder」は多次元にもできる
import os os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3' import tensorflow.compat.v1 as tf tf.disable_v2_behavior() # placeholderを使って、先に関数を組み立て # この時点ではまだxには値は代入されていない # またxのサイズは[None,3]を指定し、N行×3列のデータを後からdictionaryで指定可能に x = tf.placeholder("float", [None,3]) y = x * 3 with tf.Session() as session: # 関数「y = x * 2」に代入するxの配列を定義 x_array = [[1, 2, 3], [4, 5, 6],] result = session.run(y, feed_dict={x: x_array}) print(result)
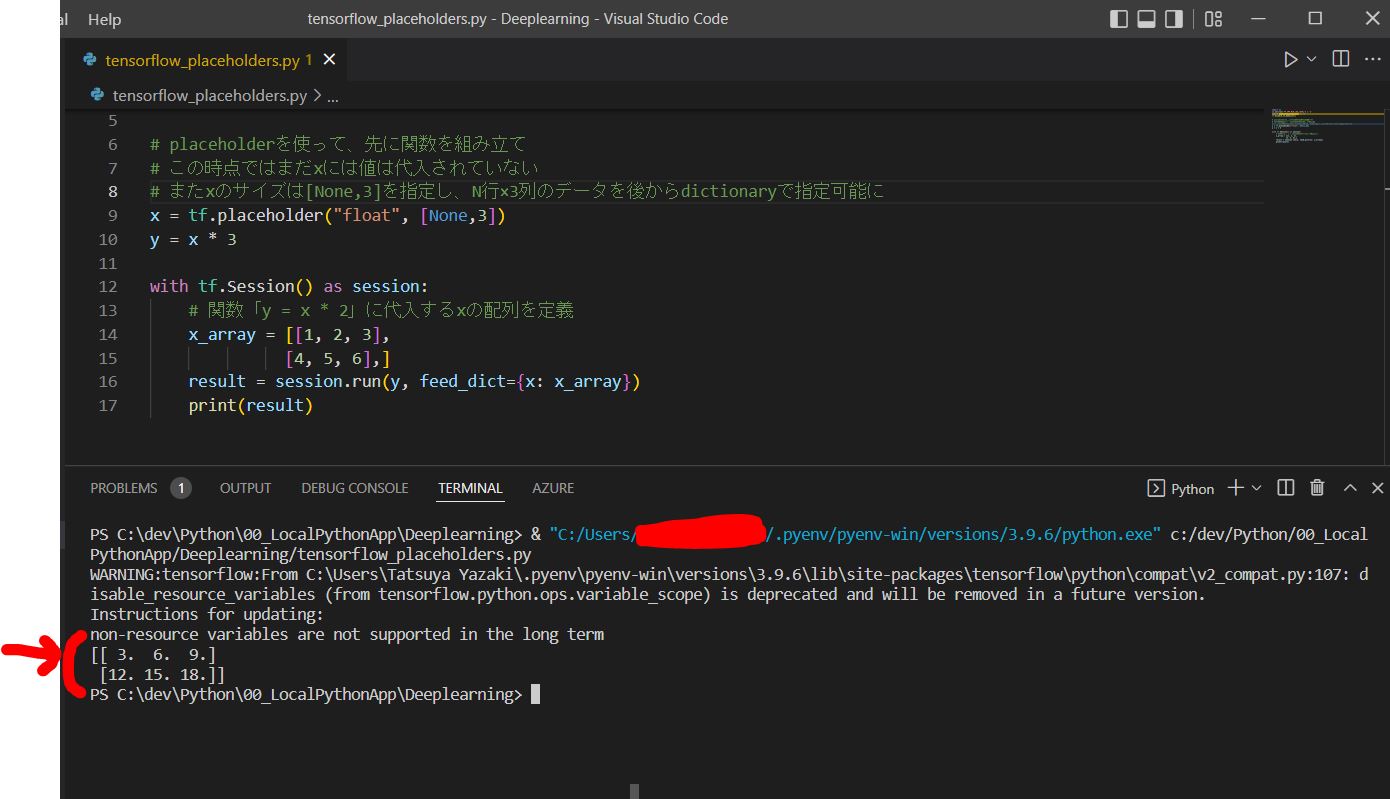
(1-3) Variableとの違いは?
●tf.Variable
●tf.placeholder
(1-4) TensorFlow 2.0以降における置きかえ
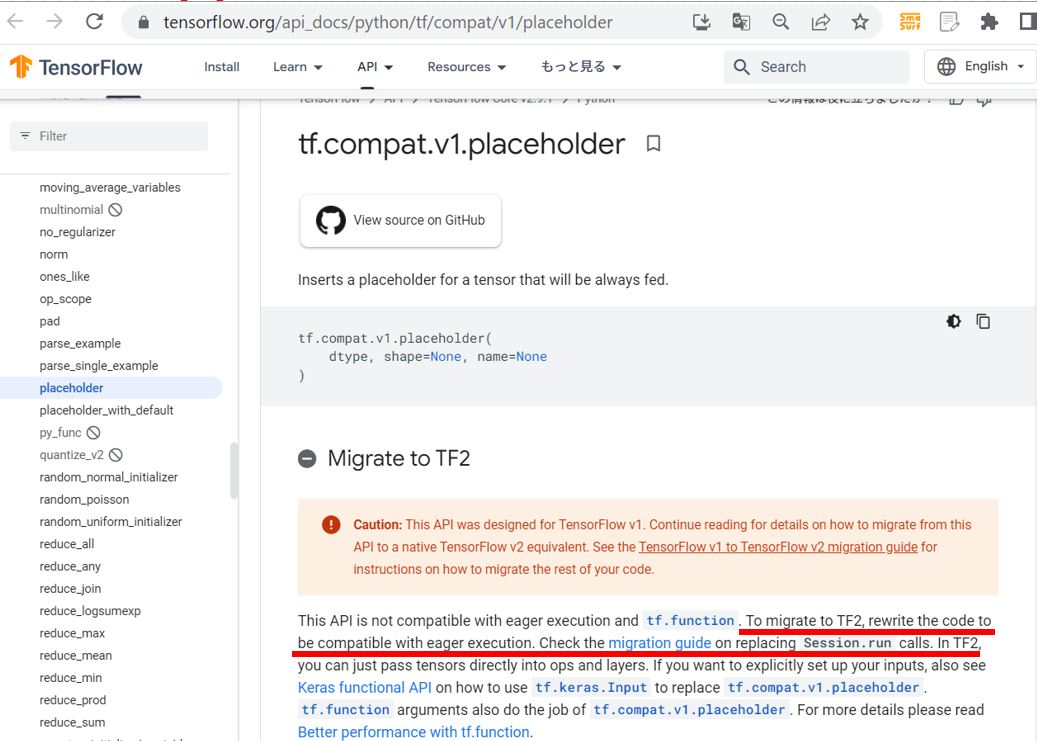
import os os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3' import tensorflow as tf @tf.function def f(x): y = x * 3 return y def main(): # 関数「y = x * 2」に代入するxの配列を定義 x_array = tf.constant([[1, 2, 3],[4, 5, 6],]) y = f(x_array) print(y) if __name__ == "__main__": main()
tf.Tensor( [[ 3 6 9] [12 15 18]], shape=(2, 3), dtype=int32)
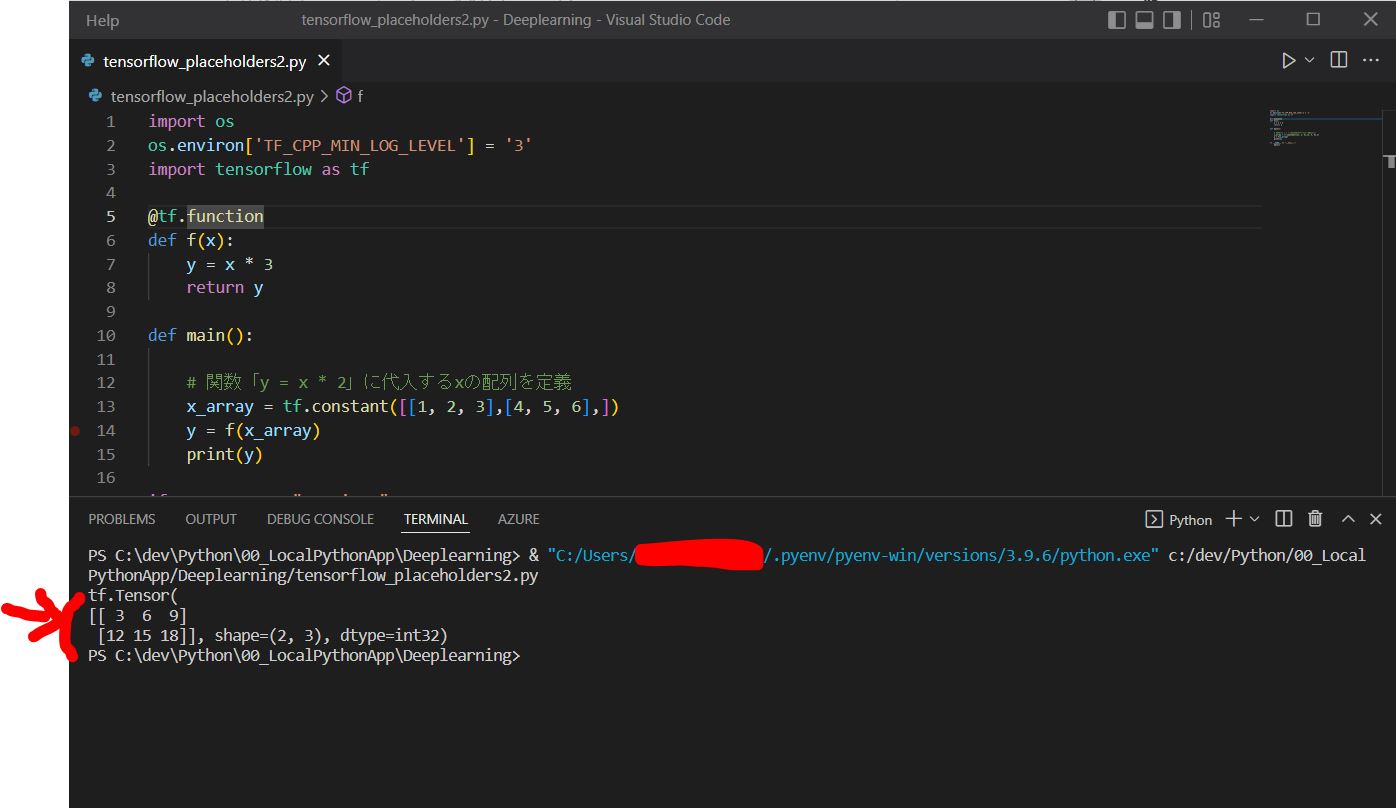