<目次>
(1) GDBの使い方をC++のプログラムのデバッグを例にご紹介
(1-1) GDBのデバッグのシナリオ概要(例)
(1-2) GDBのデバッグ手順(例)
(1-3) その他の基本的なコマンド
(1-4) エラー対応:Missing separate debuginfos, use: debuginfo-install
(1) GDBの使い方をC++のプログラムのデバッグを例にご紹介
本記事ではUnix系で使用するC++のデバッガであるGDB について、その基本的な使い方を実際の例を使ってご紹介いたします。
(1-1) GDBのデバッグのシナリオ概要(例)
(サンプル)
#include <iostream> #include <cmath> using namespace std; int CalcPow(int n) { int powResult = 0; powResult = powResult * n * n; return powResult; } double CalcPowSum(int n) { double powSum = 0.0; for (int i = 1; i <= n; i++) { powSum += 1.0 / CalcPow(n); } return powSum; } int main() { cout << "[INPUT] Enter value of n : "; int n; cin >> n; cout << endl; double powsum = CalcPowSum(n); cout << "[RESULT] The value of PowSum is : " << powsum << endl; }
(1-2) GDBのデバッグ手順(例)
●STEP0:前提条件
$ gcc –version $ g++ --version $ make –version
(図130)コマンドの出力例
●STEP1:プログラムの入手
#include <iostream> #include <cmath> using namespace std; int CalcPow(int n) { int powResult = 0; powResult = n * n; return powResult; } double CalcPowSum(int n) { double powSum = 0.0; for (int i = 1; i <= n; i++) { powSum += 1.0 / CalcPow(n); } return powSum; } int main() { cout << "[INPUT] Enter value of n : "; int n; cin >> n; cout << endl; double powsum = CalcPowSum(n); cout << "[RESULT] The value of PowSum is : " << powsum << endl; }
↓
(図131)②
●STEP2:コンパイル&実行結果の確認
$ g++ -g rainbow.cpp -o rainbow $ ./rainbow
(図132)
●STEP3:デバッガの起動
$ gdb rainbow
(コマンド実行結果)例
GNU gdb (GDB) Red Hat Enterprise Linux 7.6.1-114.el7 Copyright (C) 2013 Free Software Foundation, Inc. License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html> This is free software: you are free to change and redistribute it. There is NO WARRANTY, to the extent permitted by law. Type "show copying" and "show warranty" for details. This GDB was configured as "x86_64-redhat-linux-gnu". For bug reporting instructions, please see: <http://www.gnu.org/software/gdb/bugs/>... Reading symbols from /tmp_rainbow/rainbow...done. (gdb)
(図133)
●STEP4ブレークポイントの設置
(gdb) b 27
Breakpoint 1 at 0x400969: file rainbow.cpp, line 27.
int powsum = CalcPowSum(n);
●STEP5:デバッグ実行の開始
(gdb) run
Starting program: /tmp_rainbow/rainbow [INPUT] Enter value of n :
[INPUT] Enter value of n : 4 ↓ Breakpoint 1, main () at broken.cpp:27 27 int powsum = CalcPowSum(n);
●STEP6:関数内にステップイン&調査
(gdb) step
CalcPowSum (n=4) at rainbow.cpp:13 13 double powSum = 0.0;
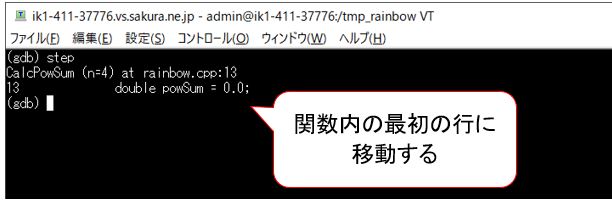
・②更にステップイン
「next」と「n」は同じ意味で、いずれもステップオーバーのコマンドです(関数にステップインせずに次に進む)。また「s」は「step」と同じ意味でステップインします。
なので、次の「next」⇒「n」で「CalcPowSum」関数内で更に次へと移動し、「s」で「CalcPow」内に更にステップインします。
(コマンド&実行結果)
(gdb) next 14 for (int i = 1; i <= n; i++) { (gdb) n 15 powSum += 1.0 / CalcPow(n); (gdb) s CalcPow (n=4) at rainbow.cpp:7 7 int powResult = 0; (gdb)
(図137)
●STEP7:変数の値の確認
(gdb) n 8 powResult = powResult * n * n; (gdb) n 9 return powResult; (gdb) print powResult $1 = 0 (gdb) quit
(図138)
(gdb) n 8 powResult = n * n; (gdb) n 9 return powResult; (gdb) print powResult $1 = 16 (gdb)
(図139)
(1-3) その他の基本的なコマンド
b [設置行番号] | ●ブレークポイントの設置 (例) 「b 21」で21行目にブレークポイントを設置する。 |
run | ●デバッグ実行 プログラムのデバッグ実行 |
step (短縮形=s) |
●ステップイン ・関数にステップインし、その関数内の最初のステートメントに移動します。 ・関数が無い場面で実行すると、nextと同じ挙動になります。 (例) main 21行目で「step」⇒CalcPowSumにステップイン |
next (短縮形=n) |
●ステップオーバー ・関数にステップイン「せずに」、次のステートメントに移動します。 |
backtrace (短縮形=bt) |
●バックトレース 実行中のスレッドにてコールされた関数の一覧です。これを確認する事で、現在地点にどのように到達したか?の流れを把握する事ができます。 |
繰り返し | ●コマンド繰り返し ・前と同じコマンドを繰り返す場合、Enterキーを押下するだけで繰り返しができます。 |
●変数の値を表示 ・「print [変数名]」で変数の名前を表示します。 |
|
quit | ●デバッガの終了 |
(1-4) エラー対応:Missing separate debuginfos, use: debuginfo-install
●事象
●対処

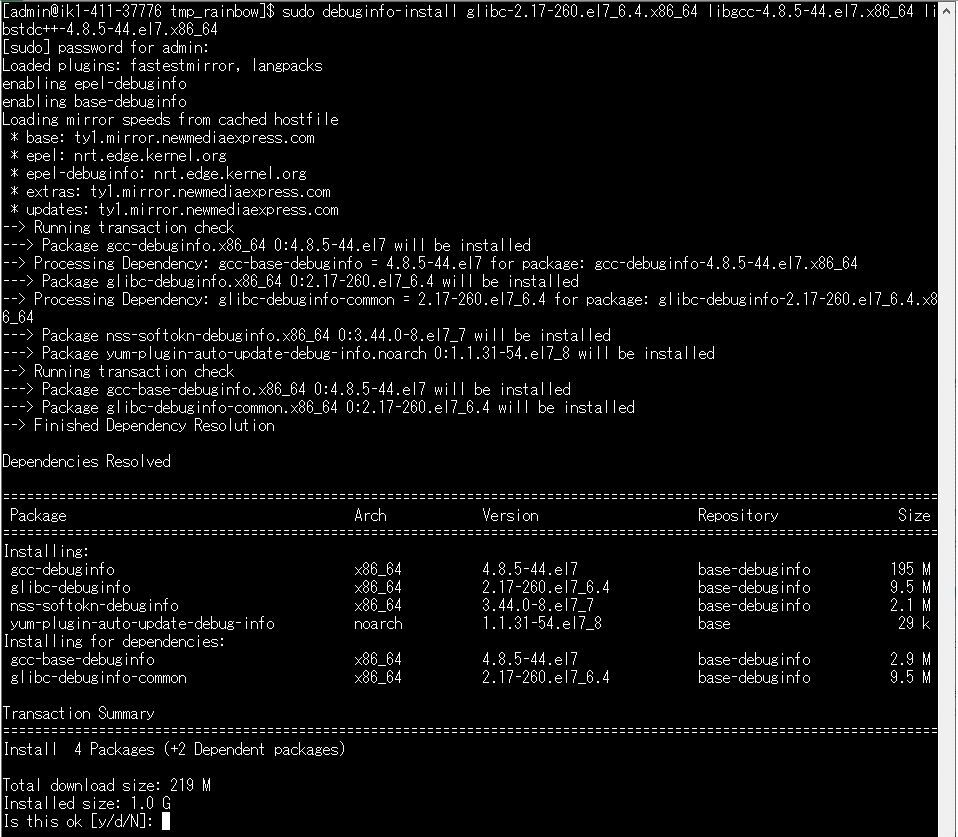
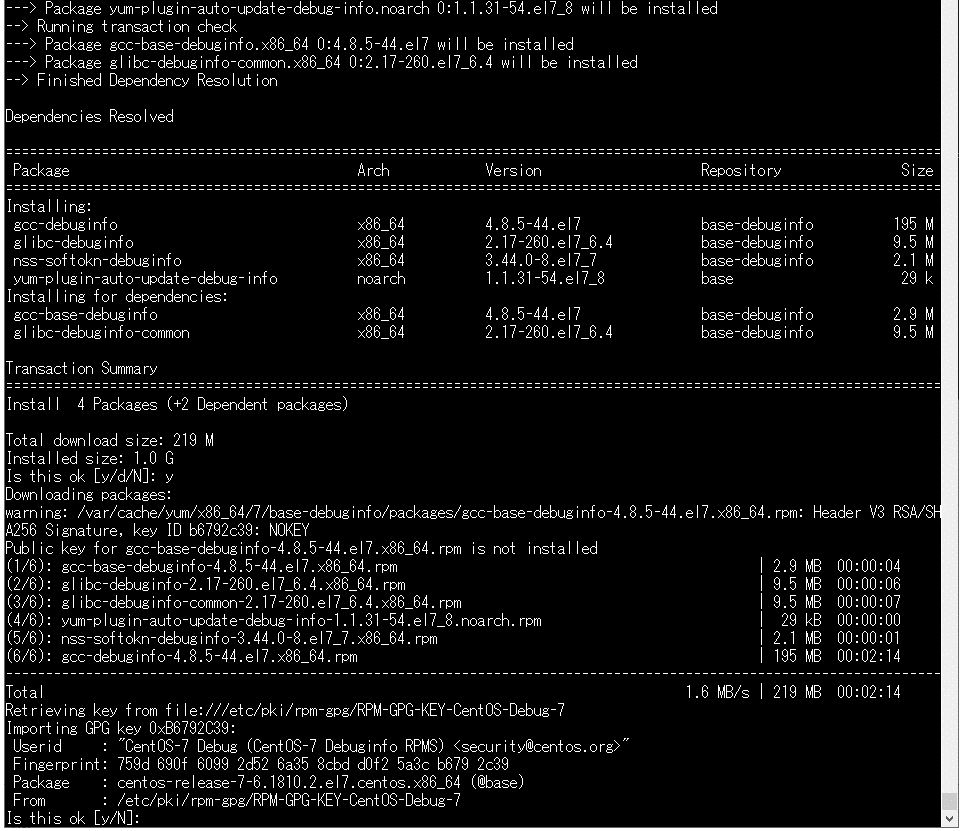
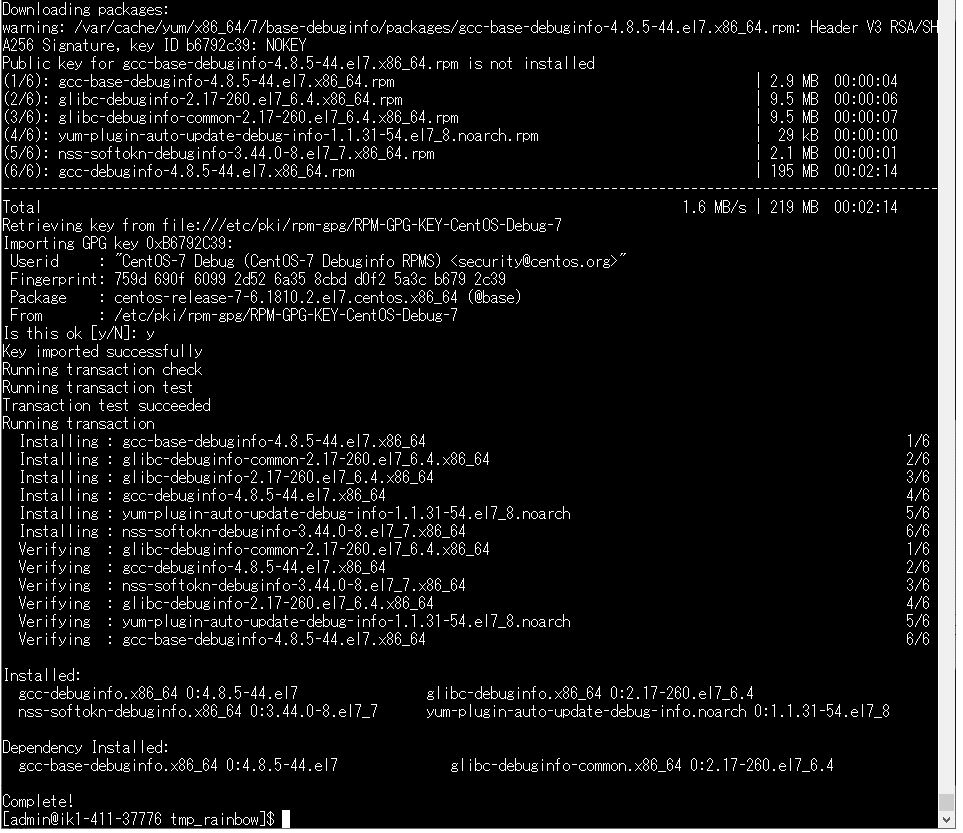