<目次>
(1) C++のマルチスレッド処理のサンプルや概要について
(1-1) C++のマルチスレッドの概要
(1-2) 構文1:関数ポインタ式
(1-3) 構文1(例):関数ポインタ式(シングル)
(1-4) 構文1(例):関数ポインタ式(マルチ)
(1-5) 構文2:関数オブジェクト式
(1-6) 構文2(例):関数オブジェクト式(シングル)
(1-7) エラー対処
(1) C++のマルチスレッド処理のサンプルや概要について
(1-1) C++のマルチスレッドの概要
(1-2) 構文1:関数ポインタ式
std::thread スレッド名(関数名, 引数1, 引数2, ・・・);
スレッド名.join();
(1-3) 構文1(例):関数ポインタ式(シングル)
#include <iostream> #include <thread> using namespace std; //# スレッドの中で呼び出す関数 void hoge(int Z) { cout << Z << endl; } int main() { //# スレッド起動(関数ポインタをCallableとして起動) thread thr1(hoge, 999); //# th1の完了を待つ thr1.join(); return 0; }
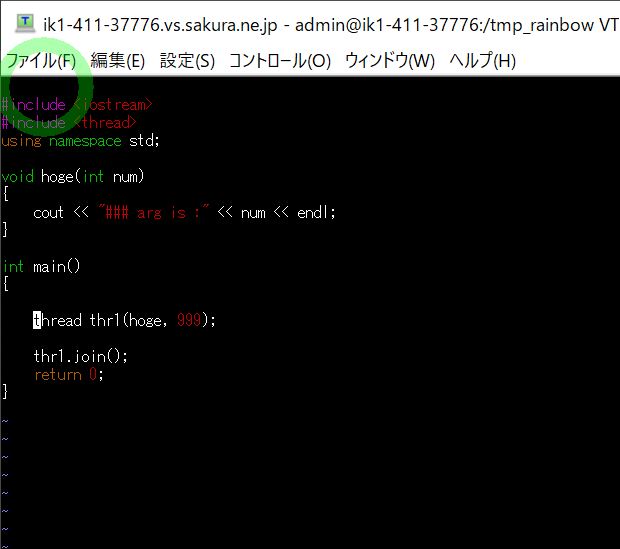
●コンパイル&実行
# コンパイル $ g++ -g -o ./[ご自身のプログラム名] ./[ご自身のプログラム名].cpp -pthread -std=c++11 # 実行 $ ./[ご自身のプログラム名]
(図122)
(1-4) 構文1(例):関数ポインタ式(マルチ)
#include <iostream> #include <thread> using namespace std; //# スレッドの中で呼び出す関数 void hoge(int Z, int thrNo) { cout<<"### THREAD#"<<thrNo<<" START : "; for(int i=0; i<Z; i++){ cout << i << " "; } cout<<endl; } int main() { //# スレッド起動(関数ポインタをCallableとして起動) thread thr1(hoge, 10, 1); thread thr2(hoge, 20, 2); thread thr3(hoge, 30, 3); //# thNの完了を待つ thr1.join(); thr2.join(); thr3.join(); return 0; }
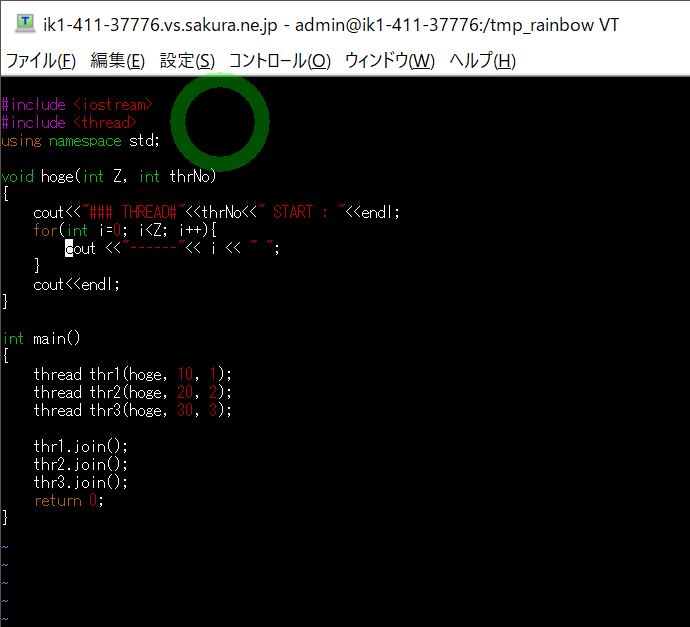
●コンパイル&実行
# コンパイル $ g++ -g -o ./[ご自身のプログラム名] ./[ご自身のプログラム名].cpp -pthread -std=c++11 # 実行 $ ./[ご自身のプログラム名]
(1-5) 構文2:関数オブジェクト式
std::thread スレッド名(関数オブジェクト名, 引数1, 引数2, ・・・);
(1-6) 構文2(例):関数オブジェクト式(シングル)
#include <iostream> #include <thread> using namespace std; //# スレッドの中で呼び出す関数 // A callable object class thr_obj { public: void operator()(int Z,int thrNo) { cout<<"### THREAD#"<<thrNo<<" START : "; for (int i = 0; i < Z; i++){ cout << i << " "; } cout<<endl; } }; int main() { //# スレッド起動(関数ポインタをCallableとして起動) thread thr2(thr_obj(), 20, 2); //# thNの完了を待つ thr2.join(); return 0; }
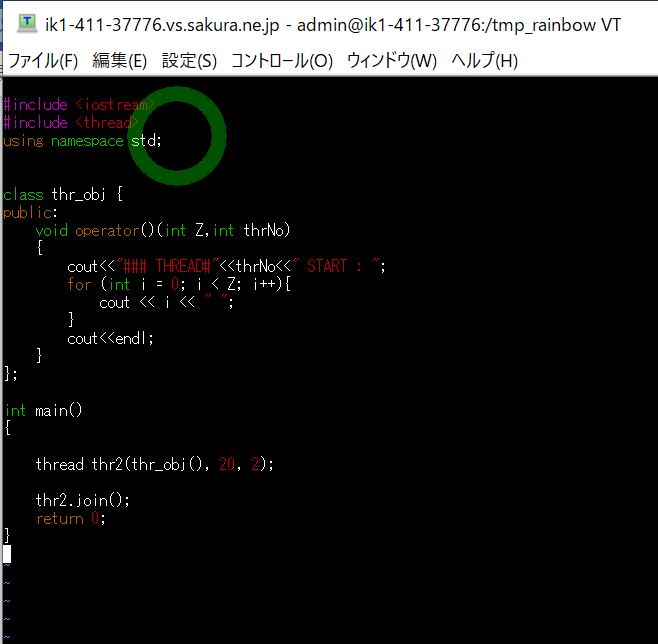
●コンパイル&実行
# コンパイル $ g++ -g -o ./[ご自身のプログラム名] ./[ご自身のプログラム名].cpp -pthread -std=c++11 # 実行 $ ./[ご自身のプログラム名]
(図142)
(1-7) エラー処理
●エラー(実行時)
terminate called after throwing an instance of 'std::system_error' what(): Enable multithreading to use std::thread: Operation not permitted Aborted (core dumped)
(図151)
●対処
コンパイル時のコマンドに「-pthread」を追記して、pthreadをリンクさせます。
# Before g++ -g -o ./thread_single1 ./thread_single1.cpp -std=c++11 # After g++ -g -o ./thread_single1 ./thread_single1.cpp -pthread -std=c++11
(図152)